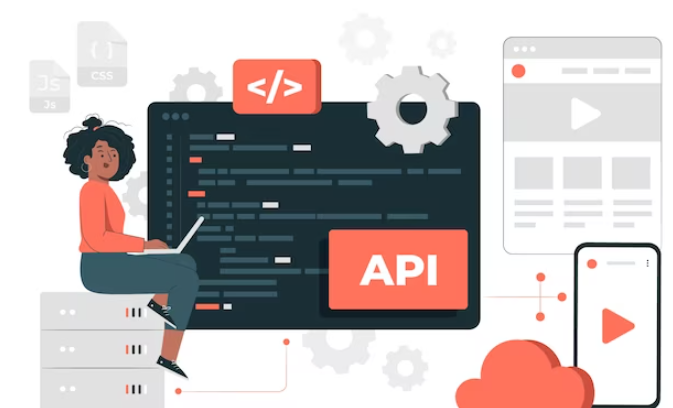
Introduction
The term REST which is short for Representational State Transfer, is a concept introduced by Roy Thomas Fielding in 2000. It serves as a visualization of an architectural style for networked systems. Unlike a physical structure, REST represents a set of design principles.
In a REST-based architecture, the central idea is to consider everything as a resource. These REST resources are essentially the data entities on which we intend to perform various operations.
In this architectural style, two key components come into play:
- REST Server: This component provides access to the resources, acting as a gateway for interactions.
- REST Client: The client, on the other hand, is responsible for accessing and making modifications to these resources.
Each of these resources is uniquely identified by a global address known as a Uniform Resource Identifier (URI) or Uniform Resource Locator (URL). Think of this as the resource’s unique address on the web.
The significance of REST APIs in the world of development cannot be overstated. They provide a framework for creating efficient, scalable, and standardized web services, making it easier for developers to build powerful and interconnected applications.
In this tutorial, we will explore ten valuable tips and tricks that developers can harness to enhance their REST API development experience.
What is an API?
The term API, an acronym for Application Programming Interface, signifies a means by which two or more applications can communicate with each other utilizing a universally recognized set of protocols. In the context of APIs, the term “Application” encompasses any software with well-defined functions, while “Interface” signifies a service contract outlining how two entities communicate through the exchange of requests and responses.
As previously discussed, we introduced two essential terms: “Client” and “Server.” API architecture is usually explained in terms of client and server. The application initiating the request is typically referred to as the “Client,” while the application providing the response is known as the “Server.”
Types of API
APIs come in various types based on how and where they are used:
- Private APIs: These are like secret tools within a company. They’re used to connect different systems and share data within the business.
- Public APIs: These are open to everyone. Anyone can use them, although sometimes there might be rules or costs involved.
- Partner APIs: These are for special friends! Only authorized external developers can use them, and they’re usually meant for business partnerships.
- Composite APIs: Think of these as combining different tools to do something big. They blend two or more APIs to handle complex tasks or needs.
APIs can operate in various modes, and their functionality often depends on the purpose and timing of their creation. However, for the duration of this tutorial, we will concentrate specifically on REST APIs. Now, let’s explore several different types of APIs below
- SOAP APIs: These APIs utilize the Simple Object Access Protocol (SOAP), where client and server communication involves the exchange of XML-based messages. SOAP represents a less flexible API technology that was more prevalent in earlier years.
- RPC APIs: These APIs are known as Remote Procedure Calls (RPC). In RPC, the client initiates the execution of a function or procedure on the server, and subsequently, the server returns the resulting output to the client.
- WebSocket APIs: WebSocket API represents a contemporary approach to web API development that leverages JSON objects for data transmission. Distinguishing itself by facilitating two-way communication between client applications and servers, WebSocket API allows servers to transmit callback messages to connected clients, thereby enhancing efficiency in comparison to traditional REST APIs.
- REST APIs: Among the APIs prevalent on the web today, REST APIs stand out as the most popular and versatile. In this paradigm, clients transmit requests to servers in the form of data, which the server then utilizes to initiate internal processes, eventually sending the resulting output data back to the client. In the subsequent sections, we will delve deeper into the intricacies of REST APIs.
Fundamentals of REST API
REST introduces a collection of operations such as GET, PUT, DELETE, OPTIONS, PATCH and POST, which clients can employ to interact with server data. This interaction between clients and servers is achieved through the utilization of the HTTP protocol.
A core characteristic of REST APIs is their statelessness. In this context, statelessness implies that ‘servers do not retain client data between successive requests’. In essence, client requests to the server closely resemble the URLs entered into a web browser to access a website.
For an API to be fully considered RESTful, a web service must adhere to the following six REST architectural constraints below:
- Layered system: One of the benefits of REST APIs is layered systems. This feature allows for an organized architecture structured into hierarchical layers, where each component interacts only with the immediate layer it’s connected to, without needing awareness of the broader system.
- Stateless operations: When a client makes a request to the server in REST, it should include all the information the server needs to understand and handle it. The key point here is that the server doesn’t retain any knowledge about the client’s state.
- Client-server-based: The uniform interface in REST keeps things organized. The client deals with how things look and what the user does (like buttons and forms), while the server handles the behind-the-scenes work like storing data, managing tasks, and security. This separation means we can improve and update the client and server without affecting each other.
- Code on demand: This feature of REST APIs involves downloading and executing code, often in the form of scripts, which enhances client-side functionality. Typically, servers return resources, commonly in XML or JSON format. In some cases, servers can also send executable code to clients as needed.
- Use of uniform interface(UI): A uniform interface in REST means we have specific rules that help all parts work smoothly. Also, each resource should have a unique web address (URL) to make them easy to find.
- RESTful resource caching: In REST, when you get a response from a request, it should clearly say if you can save it for later (cacheable) or not (non-cacheable).
Moreover, every REST request should consistently consist of four essential components:
- HTTP method
- Endpoint
- Header
- Body
Tips on securing REST APIs
Security is a vital aspect when creating a REST API to ensure it meets its intended purpose. Let’s explore some best practices for designing a secure and effective RESTful API:
- Use of HTTPS: HTTPS stands for Hyper Text Transfer Protocol Secure, which secures your data when you use a web browser. It’s the safest way to send your information from your computer to a website. HTTPS keeps your data safe by turning it into secret code before sending it to the website you’re using. It plays an essential role in securing many sensitive data.
- Input Validation: Make sure to validate that all input parameters, headers and payloads sent to the API are in the right shape and follow the rules. Also, make sure that there are no harmful or bad characters hiding in the inputs. Doing these things helps to guard against attacks like SQL injection, cross-site scripting, and command tricks. It’s like locking the door to keep the bad guys out!
- Role-based access control: Role-based access control (RBAC) is a handy tool for managing who gets to see and do what in an API. Imagine it as assigning different roles to users. RBAC is like having a bouncer at the door, making sure only the right people get in and protecting your API’s resources and data from any unwanted visitors.
- Monitor your APIs for any unusual activity: Security monitoring means keeping a close eye on the data coming in and out of your API all the time. It’s like having a security guard who’s always watching for trouble. This helps spot any suspicious activities or unwanted guests right away, so you can keep your API safe and sound. One crucial part of this is checking the logs of your system.
- Rate limit implementation: Rate limits are like traffic rules for APIs. They set a cap on how many requests a client can make in a given time frame. This rule not only helps safeguard the API from being overwhelmed by excessive requests but also serves as a defence against denial-of-service (DoS) attacks.
Rate limiting is often paired with throttling, which ensures that requests are handled at a controlled pace. This dual approach ensures the API’s computing resources are managed efficiently and protected from potential abuse. - Authentication tokens: Authentication tokens are like keys that allow users to use an API. They verify a user’s identity and access rights for a specific API call. It’s similar to how your email client uses tokens to securely access your email server when you log in.
- API Keys: API keys serve as IDs for the programs or apps that make API calls. They confirm an app’s identity and whether it has the necessary access rights for a specific API request. While not as secure as tokens, they’re useful for tracking API usage.
Importance of well-documented API
Writing a comprehensive API documentation is a crucial aspect of managing APIs. It helps developers understand how to use the API effectively and provides clarity. Here are some additional reasons why:
- Clarity and Accessibility: Make your documentation easy to find and navigate, ensuring that it’s clear and understandable to developers.
- Usage Examples: Include practical examples that demonstrate how to use the API in various scenarios. Real-world examples help developers grasp concepts quickly.
- Versioning: Clearly specify the API version, as this can impact how developers interact with it. Keeping older versions available can be beneficial for users who haven’t upgraded.
- Authentication and Authorization: Explain how authentication and authorization work, including any required API keys or tokens.
- Error Handling: Provide details about possible errors and how to handle them gracefully.
- Rate Limiting: Communicate any rate limits or usage restrictions to prevent misuse.
- Change Log: Keep a change log to inform developers of updates and modifications to the API.
- Community Engagement: Encourage developers to provide feedback and ask questions, fostering a sense of community around your API.
Conclusion
In conclusion, as you delve into the world of REST API integration, remember that it’s not just about connecting applications – it’s about creating seamless experiences for your users. At Remita, we take pride in offering a robust and secure API that can elevate your solutions to new heights. Whether you’re looking for easy authentication methods or top-notch security features, our API has got you covered.
By following best practices, embracing security measures, and harnessing the power of RESTful architecture, you can unlock a world of possibilities for your ventures. With Remita’s API, you’re not just integrating – you’re innovating.
So, let’s embark on this journey together, where your aspirations meet the excellence of Remita’s API. Your success is our priority, and we’re here to help you shine in the ever-evolving landscape of financial technology. Let’s make your dreams a reality “…because payment should be easy!”